현대 그룹에서 만든 Soofteer에서 최근 알고리즘 문제를 풀어봤다. 프로그래머스를 주로 풀면서 느꼈던 차이점은 프로그래머스에서는 입력 값을 직접 받지는 않는 반면에 소프티어에서는 직접 입력 값을 받아서 출력하는식으로 문제를 해결해야 한다.
그래서 뭐 하나만 계속 하는것보다는 프로그래머스와 소프티어 둘 다 활용해보면서 입력, 출력 문제도 연습하고, 메서드 파라미터를 활용하여 리턴 값을 가져오는 연습도 해보는 것이 좋다고 느꼈다.
평소에 코드 작성 연습을 IntelliJ IDEA에서 했었는데 자동 완성 기능에 익숙해져서 그런지 소프티어 IDE에서만 코드를 작업하는 것이 조금 힘들게 느껴진 부분도 있었다. 예를 들어 IntelliJ에서는 sout 하고 엔터를 치면 System.out.println이 바로 쳐지지만 소프티어 IDE에서는 그렇지 않았다. IDEA에 의존하지 않고 코드를 작성하는 연습이 필요할 것 같다.
소프티어에서 Level 2에 해당하는 문제들인 회의실 예약, 전광판, 비밀 메뉴, GBC, 지도 자동 구축, 장애물 인식 프로그램, 8단 변속기, 바이러스, 금고털이를 풀어보면서 느꼈던 점은 같은 Level 2여도... 엄청난 차이가 있는 거 같다.
GBC, 회의실 예약 같은 문제는 시간이 생각보다 정말 많이 걸렸다. 출력하는 방법에 미숙하기도 했고, 반복문이 많아지고, 어떤 자료구조를 사용해야 효율적인지 잘 모르겠던 부분이 많았다. 하지만 소프티어의 경우 메모리 제한은 생각보다 넉넉하게 제한하고 있는 것 같아서 메모리가 초과되는 경우는 거의 없었다.
사실 리펙토링도 많이 중요하지만...일단 실행이 되는 것이 우선이기 때문에 코드가 작동되는 것에 먼저 관점을 두고 공부를 해야할 것 같다. Java는 정말...활용도가 엄청난 거 같다. 대신 아는 만큼 더 효율적으로 사용할 수 있다는 것...
금고털이
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String[] firstInputData = reader.readLine().split(" ");
int W = Integer.parseInt(firstInputData[0]);
int N = Integer.parseInt(firstInputData[1]);
List<Stone> list = new ArrayList();
for (int i = 0; i < N; i++) {
String[] stoneData = reader.readLine().split(" ");
list.add(new Stone(Integer.parseInt(stoneData[0]), Integer.parseInt(stoneData[1])));
}
Collections.sort(list);
int result = 0;
int stoneIndex = 0;
while (true) {
Stone stone = list.get(stoneIndex);
if (stone.M < W) {
W -= stone.M;
result += stone.M * stone.P;
stoneIndex++;
continue;
}
result += W * stone.P;
break;
}
System.out.println(result);
}
}
class Stone implements Comparable<Stone> {
int M;
int P;
public Stone(int M, int P) {
this.M = M;
this.P = P;
}
@Override
public int compareTo(Stone obj) {
return obj.P - this.P;
}
}
바이러스
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer tokenizer = new StringTokenizer(reader.readLine());
long K = Integer.parseInt(tokenizer.nextToken());
long P = Integer.parseInt(tokenizer.nextToken());
int N = Integer.parseInt(tokenizer.nextToken());
for (int i = 1; i <= N; i++) {
K = K * P % 1000000007;
}
System.out.println(K);
}
}
8단 변속기
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String inputData = reader.readLine();
if (inputData.equals("1 2 3 4 5 6 7 8")) {
System.out.println("ascending");
} else if (inputData.equals("8 7 6 5 4 3 2 1")) {
System.out.println("descending");
} else {
System.out.println("mixed");
}
}
}
장애물 인식 프로그램
import java.util.*;
import java.io.*;
public class Main
{
static int count = 0;
public static void main(String args[]) throws IOException
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int N = Integer.parseInt(br.readLine());
int[][] graph = new int[N][N];
List<Integer> result = new ArrayList<>();
for (int i = 0; i < N; i++) {
String[] data = br.readLine().split("");
for (int j = 0; j < N; j++) {
graph[i][j] = Integer.parseInt(data[j]);
}
}
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
count = 0;
if (graph[i][j] == 0) {
continue;
}
dfs(i, j, graph, N);
result.add(count);
}
}
Collections.sort(result);
System.out.println(result.size());
result.stream().forEach(System.out::println);
}
public static void dfs(int i, int j, int[][] graph, int N) {
if (i < 0 || j < 0 || i >= N || j >= N) {
return;
}
if (graph[i][j] == 0) {
return;
}
if (graph[i][j] == 1) {
graph[i][j] = 0;
count++;
dfs(i, j + 1, graph, N);
dfs(i, j - 1, graph, N);
dfs(i + 1, j, graph, N);
dfs(i - 1, j, graph, N);
}
}
}
지도 자동 구축
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
int N = Integer.parseInt(reader.readLine());
int result = getResult(N);
System.out.println(result * result);
}
public static int getResult(int N) {
if (N == 0) {
return 2;
}
return getResult(N - 1) + (int)Math.pow(2, N - 1);
}
}
GBC
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer tokenizer = new StringTokenizer(reader.readLine());
int n = Integer.parseInt(tokenizer.nextToken());
int m = Integer.parseInt(tokenizer.nextToken());
Section nSection = new Section();
int before = 0;
for (int i = 0; i < n; i++) {
tokenizer = new StringTokenizer(reader.readLine());
nSection.scope.add(before + Integer.parseInt(tokenizer.nextToken()));
nSection.speed.add(Integer.parseInt(tokenizer.nextToken()));
before = nSection.scope.get(i);
}
Section mSection = new Section();
before = 0;
for (int i = 0; i < m; i++) {
tokenizer = new StringTokenizer(reader.readLine());
mSection.scope.add(before + Integer.parseInt(tokenizer.nextToken()));
mSection.speed.add(Integer.parseInt(tokenizer.nextToken()));
before = mSection.scope.get(i);
}
int result = 0;
before = 0;
for (int i = 0; i < n; i++) {
int sectionLimitSpeed = nSection.speed.get(i);
int sectionMaxSpeed = mSection.getMaxSpeed(before, nSection.scope.get(i));
result = Math.max(result, sectionMaxSpeed - sectionLimitSpeed);
before = nSection.scope.get(i) + 1;
}
System.out.println(result);
}
}
class Section {
List<Integer> scope = new ArrayList<>();
List<Integer> speed = new ArrayList<>();
public int getMaxSpeed(int start, int end) {
int s = 0;
for (int i = start; i <= end; i++) {
s = Math.max(s, getSpeed(i));
}
return s;
}
public int getSpeed(int value) {
int s = 0;
for (int i = 0; i < scope.size(); i++) {
if (value <= scope.get(i)) {
s = speed.get(i);
break;
}
}
return s;
}
}
[21년 재직자 대회 예선] 비밀 메뉴
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String[] inputData = reader.readLine().split(" ");
int M = Integer.parseInt(inputData[0]);
int N = Integer.parseInt(inputData[1]);
int K = Integer.parseInt(inputData[2]);
String[] SecretCode = reader.readLine().split(" ");
String[] MyCode = reader.readLine().split(" ");
boolean isSecret = false;
for (int i = 0; i < MyCode.length; i++) {
if (MyCode.length - i < SecretCode.length || isSecret) {
break;
}
if (MyCode[i].equals(SecretCode[0])) {
isSecret = Arrays.equals(Arrays.copyOfRange(MyCode, i, i + SecretCode.length), SecretCode);
}
}
if (isSecret) {
System.out.println("secret");
} else {
System.out.println("normal");
}
}
}
[21년 재직자 대회 예선] 전광판
import java.util.*;
import java.io.*;
public class Main
{
private static final List<int[]> digitInfo = new ArrayList<>() {{
add(new int[]{1, 1, 1, 0, 1, 1, 1});
add(new int[]{0, 0, 1, 0, 0, 1, 0});
add(new int[]{1, 0, 1, 1, 1, 0, 1});
add(new int[]{1, 0, 1, 1, 0, 1, 1});
add(new int[]{0, 1, 1, 1, 0, 1, 0});
add(new int[]{1, 1, 0, 1, 0, 1, 1});
add(new int[]{1, 1, 0, 1, 1, 1, 1});
add(new int[]{1, 1, 1, 0, 0, 1, 0});
add(new int[]{1, 1, 1, 1, 1, 1, 1});
add(new int[]{1, 1, 1, 1, 0, 1, 1});
}};
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
int T = Integer.parseInt(reader.readLine());
for (int i = 0; i < T; i++) {
StringTokenizer tokenizer = new StringTokenizer(reader.readLine());
int firstValue = Integer.parseInt(tokenizer.nextToken());
int secondValue = Integer.parseInt(tokenizer.nextToken());
System.out.println(testCase(firstValue, secondValue));
}
}
public static int testCase(int firstValue, int secondValue) {
int result = 0;
List<Integer> firstValueDigit = getDigitInfo(firstValue);
List<Integer> secondValueDigit = getDigitInfo(secondValue);
for (int i = 0; i < 5; i++) {
result += getChangeCount(firstValueDigit.get(i), secondValueDigit.get(i));
}
return result;
}
public static int getChangeCount(int x, int y) {
if (x == -1 && y == -1) {
return 0;
}
if (x == -1) {
return (int)Arrays.stream(digitInfo.get(y)).filter(data -> data == 1).count();
}
if (y == -1) {
return (int)Arrays.stream(digitInfo.get(x)).filter(data -> data == 1).count();
}
int changeCount = 0;
int[] xInfo = digitInfo.get(x);
int[] yInfo = digitInfo.get(y);
for (int i = 0; i < 7; i++) {
if (xInfo[i] != yInfo[i]) {
changeCount++;
}
}
return changeCount;
}
public static List<Integer> getDigitInfo(int value) {
List<Integer> info = new ArrayList<>();
for (int i = 0; i < 5; i++) {
info.add(value / 10000);
info.add(value % 10000 / 1000);
info.add(value % 1000 / 100);
info.add(value % 100 / 10);
info.add(value % 10);
}
int length = String.valueOf(value).length();
for (int i = 0; i < 5 - length; i++) {
info.set(i, -1);
}
return info;
}
}
[21년 재직자 대회 예선] 회의실 예약
import java.util.*;
import java.io.*;
public class Main
{
public static void main(String args[]) throws IOException
{
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String[] firstInputData = reader.readLine().split(" ");
int N = Integer.parseInt(firstInputData[0]);
int M = Integer.parseInt(firstInputData[1]);
Map<String, ConferenceRoom> conferenceRoom = new HashMap<String, ConferenceRoom>();
for (int i = 0; i < N; i++) {
String name = reader.readLine();
conferenceRoom.put(name, new ConferenceRoom(name));
}
for (int i = 0; i < M; i++) {
String[] conferenceData = reader.readLine().split(" ");
String name = conferenceData[0];
int start = Integer.parseInt(conferenceData[1]);
int end = Integer.parseInt(conferenceData[2]);
List<Boolean> currentAvailable = conferenceRoom.get(name).available;
for (int j = start - 9; j <= end - 10; j++) {
currentAvailable.set(j, false);
}
}
List<String> sortedKey = new ArrayList<>(conferenceRoom.keySet());
Collections.sort(sortedKey);
for (int i = 0; i < sortedKey.size(); i++) {
System.out.println("Room " + conferenceRoom.get(sortedKey.get(i)).name + ":");
ConferenceRoom pickedConferenceRoom = conferenceRoom.get(sortedKey.get(i));
List<Boolean> currentAvailable = pickedConferenceRoom.available;
if (!currentAvailable.contains(true)) {
System.out.println("Not available");
} else {
System.out.println(pickedConferenceRoom.getCount() + " available:");
pickedConferenceRoom.printCurrentAvailable();
}
if (i != sortedKey.size() - 1) {
System.out.println("-----");
}
}
}
}
class ConferenceRoom {
String name;
List<Boolean> available;
List<int[]> availableTime;
public ConferenceRoom(String name) {
this.name = name;
available = new ArrayList<Boolean>();
for (int i = 0; i < 9; i++) {
available.add(true);
}
availableTime = new ArrayList<int[]>();
}
public int getCount() {
int count = 0;
boolean before = false;
int start = 0;
int end = 0;
for (int i = 0; i < available.size(); i++) {
if (!before && available.get(i)) {
start = i + 9;
before = true;
} else if (before && !available.get(i)) {
end = i + 9;
before = false;
count++;
availableTime.add(new int[]{start, end});
}
}
if (before) {
count++;
availableTime.add(new int[]{start, 18});
}
return count;
}
public void printCurrentAvailable() {
for (int[] a : availableTime) {
System.out.printf("%02d-%02d\n", a[0], a[1]);
}
}
}
정답을 맞추기 위해서 풀었던 문제들이 많았기에...코드가 가독성이 많이 떨어집니다... 그냥 기록이라도 해놓기 위해서 올려둔 것이기 때문에 불편해도 어쩔 수 없습니다. 하하하 🤓
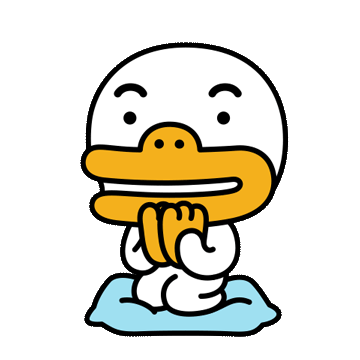
'🧑🏻💻 Dev > 알고리즘' 카테고리의 다른 글
[프로그래머스] 혼자서 하는 틱택토 Java (0) | 2023.03.20 |
---|---|
[프로그래머스] 레벨 1 문제 모두 풀고난 후 배운점 (2) | 2023.03.17 |
[프로그래머스] 시소 짝꿍 (Java) (0) | 2023.02.15 |
[Sorfteer] 인증평가(5차) 기출 - 성적 평가 (Java) (0) | 2023.02.07 |
[Sorfteer] 인증평가(5차) 기출 - 업무 처리 (Java) (0) | 2023.01.18 |